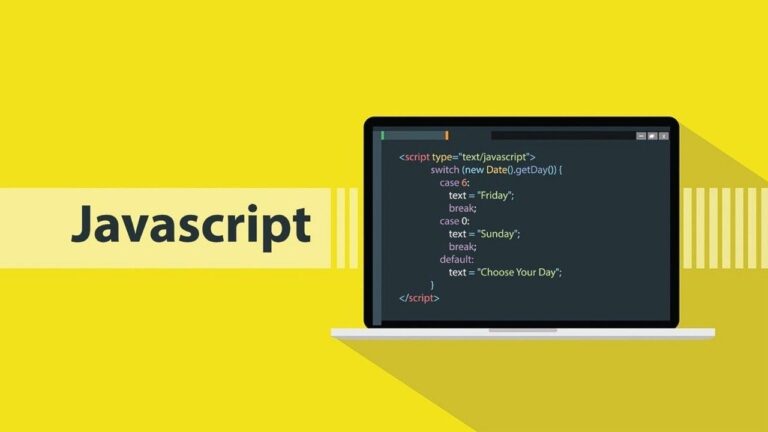
جاواسکریپت: ۶- کاندیشن ۱
شرط – Conditions
در جاواسکریپت شرط را به سه گونه میتوان نوشت.
- ?
- if
- Switch
همه آنها را باید یاد گرفت و در هر جایی آن روشی را که سادهتر است، به کار برد.
تِرنِری – Ternary (?)
ترنری سادهترین فرم شرط است. و چون از سه بخش تشکیل میشود، آن را ترنری به معنای سهتایی مینامند.
condition ? valueTrue : valueFalse
let x = 5;
let a = (x > 0) ? 10 : 20; // a = 10
let b = (x < 0) ? 10 : 20; // b = 20
مثال
در مثال زیر یک عدد را میگیرد و مشخص میکند مثبت است یا منفی.
<input type="text" id="in-box">
<button id="btn">OK</button>
<h1 id="out-box"></h1>
const inBox = document.getElementById("in-box");
const outBox = document.getElementById("out-box");
const btn = document.getElementById("btn");
btn.onclick = function() {
let numVal = inBox.value;
let numType = (numVal < 0) ? "negative" : "positive";
outBox.innerHTML = numType;
}
مثال
در مثال زیر یک تصویر SVG وجود دارد که از دو لایه تشکیل شده است. لایهٔ زیر، نقرهای و لایهٔ رو، زرد است. با کلیک کردن روی کلید، یک بار در میان، لایهٔ رو (که زرد رنگ است)، پنهان یا پیدا میشود.
<g id="on"> ... </g>
...
<div id="power">Power</div>
const on = document.getElementById("on");
const power = document.getElementById("power");
power.onclick = function() {
let light = on.style.display;
light = (light == "none") ? "block" : "none";
on.style.display = light;
}
if else
if (Condition) { … }
let x = 5;
let a;
if (x < 0) {
a = 1; // if true
}
// a = undefined
if (x > 0) {
a = 1; // if true
}
// a = 1
مثال
<input type="text" id="in-box">
<button id="btn">OK</button>
<h1 id="out-box"></h1>
const inBox = document.getElementById("in-box");
const outBox = document.getElementById("out-box");
const btn = document.getElementById("btn");
btn.onclick = function() {
let numVal = inBox.value;
if (numVal < 0) {
outBox.innerHTML = "Negative";
}
}
if (Condition) { … } else { … }
let x = 5;
let a;
if (x > 0) {
a = 1; // if true
} else {
a = -1; // if false
}
// a = 1
if (x < 0) {
a = 1; // if true
} else {
a = -1; // if false
}
// a = -1
مثال
<input type="text" id="in-box">
<button id="btn">OK</button>
<h1 id="out-box"></h1>
const inBox = document.getElementById("in-box");
const outBox = document.getElementById("out-box");
const btn = document.getElementById("btn");
btn.onclick = function() {
let numVal = inBox.value;
if (numVal < 0) {
outBox.innerHTML = "Negative";
} else {
outBox.innerHTML = "Positive";
}
}
if (Condition1) { … } else if (Condition2) { … } else { … }
let x = 5;
let a;
if (x > 0) {
a = 1; // if condition1 is true
} else if (x < 0) {
a = -1; // if condition2 is true
} else {
a = 0; // if all is false
}
// a = 1
مثال
<input type="text" id="in-box">
<button id="btn">OK</button>
<h1 id="out-box"></h1>
const inBox = document.getElementById("in-box");
const outBox = document.getElementById("out-box");
const btn = document.getElementById("btn");
btn.onclick = function() {
let numVal = inBox.value;
if (numVal < 0) {
outBox.innerHTML = "Negative";
} else if (numVal > 0) {
outBox.innerHTML = "Positive";
} else {
outBox.innerHTML = "Zero";
}
}
تمرین ۱
-
- این فایل را دانلود کنید.
- آن را در فولدر js1 باز کنید.
- تنها فایل script.js را میتوانید تغییر دهید.
- نام و پسورد را بگیرد و در صورت نادرست بودن هرکدام زیر خودش پیام مناسب ظاهر شود.
اسکوپ – Scope
- اسکوپ به معنای محدوده است. (تلسکوپ و مایکروسکوپ، ترکیبی از همین کلمه است.)
- اسکوپ، به بخشی از کد میگویند که بین دو علامت {…} قرار گرفته است. (نام این علامت کِرلی بِرَکِت – Curly Bracket است.)
- در جاواسکریپت توجه به اسکوپ، بسیار مهم است. چون اگر یک متغیر، در اسکوپی تعریف شده باشد، بیرون آن کار نمیکند.
گلوبال – Global
اگر یک متغیر، بیرون اسکوپها تعریف شده باشد، همه جا قابل استفاده است. و به آن، گلوبال یا سراسری می گویند.
let fruit = "apple";
alert(fruit); // apple
if (true) {
alert(fruit); // apple
}
لوکال – Local
اگر متغیر درون یک اسکوپ تعریف شده باشد، تنها درون همان اسکوپ قابل استفاده است. و به آن، لوکال یا محلی می گویند.
if (true) {
let fruit = "apple";
alert(fruit); // apple
}
alert(fruit); // undefined
متغیرهای همنام در اسکوپهای متفاوت همانند دو متغیر جداگانه کار میکنند.
let fruit = "apple";
alert(fruit); // apple
if (true) {
let fruit = "orange";
alert(fruit); // orange
}
alert(fruit); // apple
مقدار هر متغیر بیرونی در داخل اسکوپ، در دسترس است و میتوان آن را تغییر داد.
let fruit = "apple";
alert(fruit); // apple
if (true) {
fruit = "orange";
alert(fruit); // orange
}
alert(fruit); // orange
Math
آبجکت Math با مقادیر عددی کار میکند.
Math.PI = 3.141592653589793
<input type="text" id="in-box">
<button id="btn">OK</button>
<h1 id="out-box"></h1>
const inBox = document.getElementById("in-box");
const outBox = document.getElementById("out-box");
const btn = document.getElementById("btn");
btn.onclick = function() {
let d = inBox.value;
let c = d * Math.PI;
outBox.innerHTML = c;
}
Math.random( )
یک عدد رَندوم یا تصادفی بین صفر و یک [0,1) میدهد.
<button id="btn">OK</button>
<h1 id="out-box"></h1>
const outBox = document.getElementById("out-box");
const btn = document.getElementById("btn");
btn.onclick = function() {
let r = Math.random();
outBox.innerHTML = r;
}
Math.trunc(x)
بخش صحیح عدد را برمیگرداند.
<input type="text" id="in-box">
<button id="btn">OK</button>
<h1 id="out-box"></h1>
const inBox = document.getElementById("in-box");
const outBox = document.getElementById("out-box");
const btn = document.getElementById("btn");
btn.onclick = function() {
let num = inBox.value;
let i = Math.trunc(num);
outBox.innerHTML = i;
}
Math.floor(x)
نزدیکترین عدد صحیح کوچکتر یا مساوی x را برمیگرداند.
<input type="text" id="in-box">
<button id="btn">OK</button>
<h1 id="out-box"></h1>
const inBox = document.getElementById("in-box");
const outBox = document.getElementById("out-box");
const btn = document.getElementById("btn");
btn.onclick = function() {
let num = inBox.value;
let i = Math.floor(num);
outBox.innerHTML = i;
}
Math.ceil( )
نزدیکترین عدد صحیح بزرگتر یا مساوی x را برمیگرداند.
<input type="text" id="in-box">
<button id="btn">OK</button>
<h1 id="out-box"></h1>
const inBox = document.getElementById("in-box");
const outBox = document.getElementById("out-box");
const btn = document.getElementById("btn");
btn.onclick = function() {
let num = inBox.value;
let i = Math.ceil(num);
outBox.innerHTML = i;
}
Math.round(x)
نزدیکترین عدد صحیح به x را برمیگرداند.
<input type="text" id="in-box">
<button id="btn">OK</button>
<h1 id="out-box"></h1>
const inBox = document.getElementById("in-box");
const outBox = document.getElementById("out-box");
const btn = document.getElementById("btn");
btn.onclick = function() {
let num = inBox.value;
let i = Math.round(num);
outBox.innerHTML = i;
}
مقایسه نتیجه فانکشنهای بالا
x | -1 | -0.9 | -0.5 | -0.1 | 0 | 0.1 | 0.5 | 0.9 | 1 |
---|---|---|---|---|---|---|---|---|---|
trunc(x) | -1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 |
floor(x) | -1 | -1 | -1 | -1 | 0 | 0 | 0 | 0 | 1 |
ceil(x) | -1 | 0 | 0 | 0 | 0 | 1 | 1 | 1 | 1 |
round(x) | -1 | -1 | 0 | 0 | 0 | 0 | 1 | 1 | 1 |
تمرین ۲
- این فایل را دانلود کنید.
- آن را در فولدر js1 باز کنید.
- تنها فایل script.js را میتوانید تغییر دهید.
- هنگامیکه روی یکی از تصاویر زیر کلیک می کنید نام آن زیر User نوشته می شود.
- کامپیوتر به صورت رندوم یکی را انتخاب می کند و نام آن را زیر Computer می نویسد.
- و در پایان برنده یا بازنده شدن کاربر در وسط صفحه نوشته میشود.
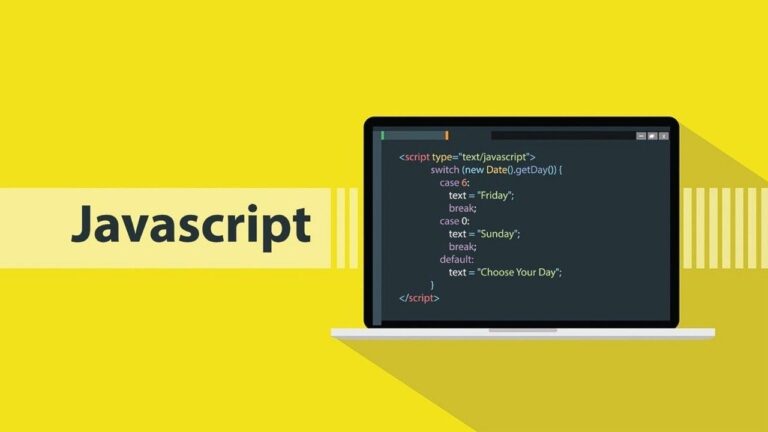
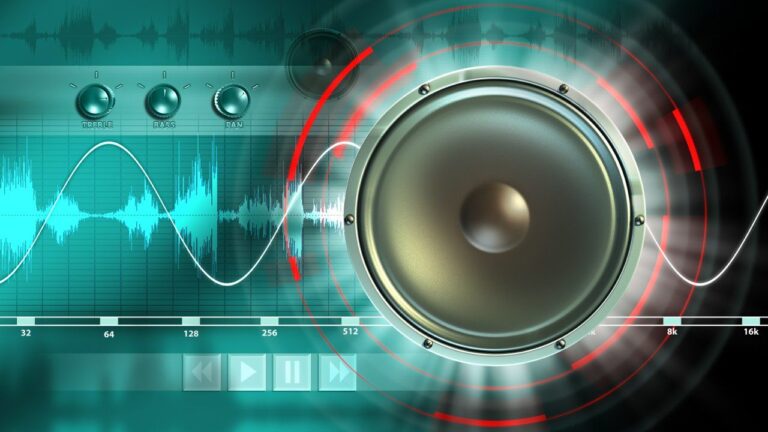
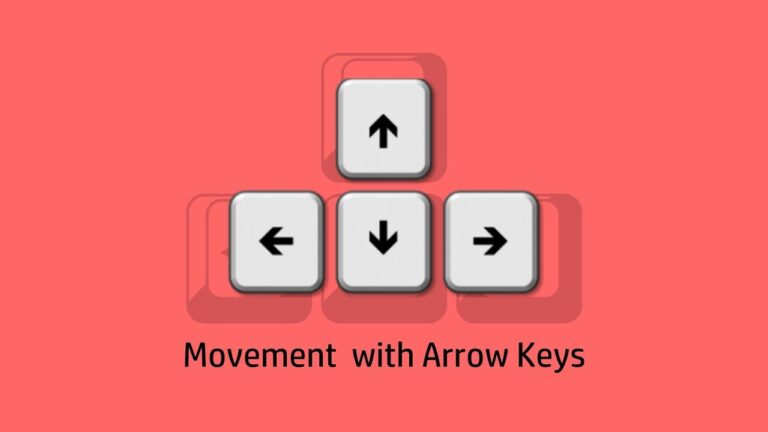